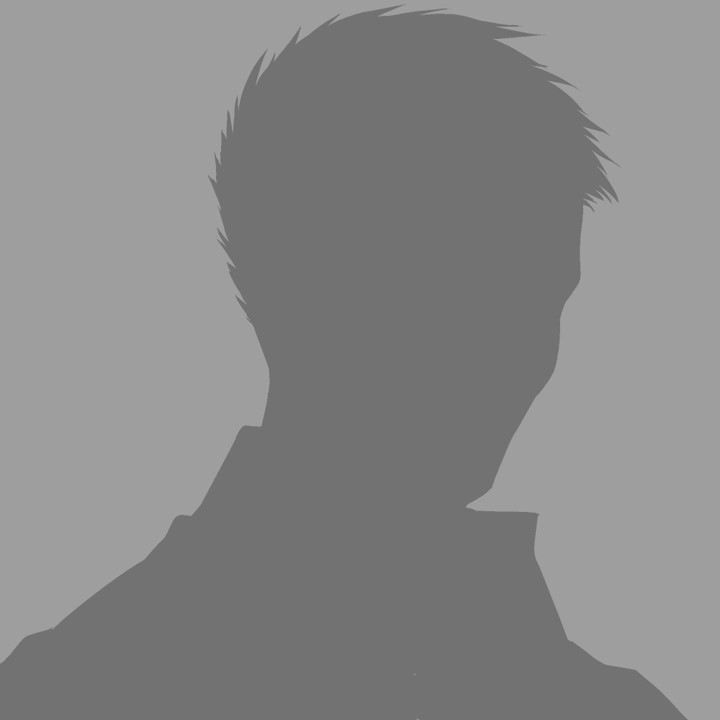
higashi
Hi, I’m higashi.
This page introduce how to display the coordinate of mouse clicked position on the image like below.
So let’s get stated !!
Necessary Libraries
★OpenCV
★Numpy
If you’ve not installed, please install first.
Sample Code of Display the Clicked Position on the Image
As shown below is the program of display the clicked position on the image.
import cv2
import numpy as np
file_name='sample.jpg'
img=cv2.imread(file_name,cv2.IMREAD_GRAYSCALE)
def click_pos(event, x, y, flags, params):
if event == cv2.EVENT_LBUTTONDOWN:
img2 = np.copy(img)
cv2.circle(img2,center=(x,y),radius=5,color=255,thickness=-1)
pos_str='(x,y)=('+str(x)+','+str(y)+')'
cv2.putText(img2,pos_str,(x+10, y+10),cv2.FONT_HERSHEY_PLAIN,2,255,2,cv2.LINE_AA)
cv2.imshow('window', img2)
cv2.imshow('window', img)
cv2.setMouseCallback('window', click_pos)
cv2.waitKey(0)
cv2.destroyAllWindows()
The contents of the implementation are as follows.
- Loading Image
- Display the Loaded Image on Window
- Get a Clicked Positional Coordinate
- Describe Marker and Coordinate on Original Image and Display Again
In the Case of Display Position by Only Mouse Moving
The positional coordinate can display not only by mouse click but also by only mouse movement as shown below.
The sample code is this.
import cv2
import numpy as np
file_name='mouse.jpg'
img=cv2.imread(file_name,cv2.IMREAD_GRAYSCALE)
def mouse_move(event, x, y, flags, params):
if event == cv2.EVENT_MOUSEMOVE:
img2 = np.copy(img)
cv2.circle(img2,center=(x,y),radius=5,color=255,thickness=-1)
pos_str='(x,y)=('+str(x)+','+str(y)+')'
cv2.putText(img2,pos_str,(30, 30),cv2.FONT_HERSHEY_PLAIN,2,255,2,cv2.LINE_AA)
cv2.imshow('window', img2)
cv2.imshow('window', img)
cv2.setMouseCallback('window', mouse_move)
cv2.waitKey(0)
cv2.destroyAllWindows()
The change is only ‘cv2.EVENT_LBUTTONDOWN’ (means left click) to ‘cv2.EVENT_MOUSEMOVE’ (means mouse move).
Please use according to the situation.
That’s all. Thank you!!
コメント